Support Vector Machines(SVM)
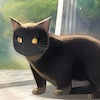
0x01 SVM
实现算法:序列最小优化(SMO)
支持向量:离分割超平面最近的那些点
优点:
- 范化错误率低
- 计算开销不大
- 结果易解释
缺点:
- 对参数调节和核函数的选择敏感
- 原始分类器需要修改才能处理多分类问题
适用数据类型:
- 数值型
- 标称型
0x02 SMO
将大优化问题分解为多个小优化问题来求解
目标是求出一系列alpha和b,一旦求出了这些alpha,就很容易计算出权重向量w并得到分隔超平面
工作原理是:每次循环中选择两个alpha进行优化处理。一旦找到一对合适的alpha,那么就增大其中一个同时减小另一个
- 简化版实现
1 | def selectJrand(i, m): |
找出哪些点是支持向量
1 | for i in range(100): |
- 完整版实现
1 | class optStruct: |
1 | for i in range(100): |
最后一行为测试结果,小于0属于-1类,大于0属于1类,等于0属于-1类
0x03 kernel
- 将数据映射到高维空间
将数据从一个特征空间转换到另一个特征空间
映射会将低维特征空间映射到高维空间
- 径向基核函数
1 | def kernelTrans(X, A, kTup): |
测试
1 | def testRbf(k1=1.3): |
0x04 实例1
基于SVM的手写数字识别
1 | def testDigits(kTup=('rbf', 10)): |
0x05 实例2
XSS Detection
0x01 数据
在github上看到https://github.com/SparkSharly/DL_for_xss 这个项目,感觉不错,学习一下,数据集项目中已经附带,就直接使用了
- eg. normal_examples.csv (20w+取部分)
- eg. xssed.csv (4W+取部分)
0x02 分词
1 | def GeneSeg(payload): |
0x03 特征
- 建立xss语义模型,构建词汇表
统计高频出现的300词构建词表
1 | words=[] |
- word2vec建模
1 | model=Word2Vec(data_set,size=embedding_size,window=skip_window,negative=num_sampled,iter=num_iter) |
空间维度设置为32维
查看建模结果,与</script>
最语义最相近的词
- 数据处理
1 | def pre_process(): |
0x04 SVM训练
通过SVM算法进行模型训练
1 | train_datas, train_labels=pre_process() |
精确率和召回率:
- Post title:Support Vector Machines(SVM)
- Post author:langu_xyz
- Create time:2019-07-22 21:00:00
- Post link:https://blog.langu.xyz/Support Vector Machines(SVM)/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.